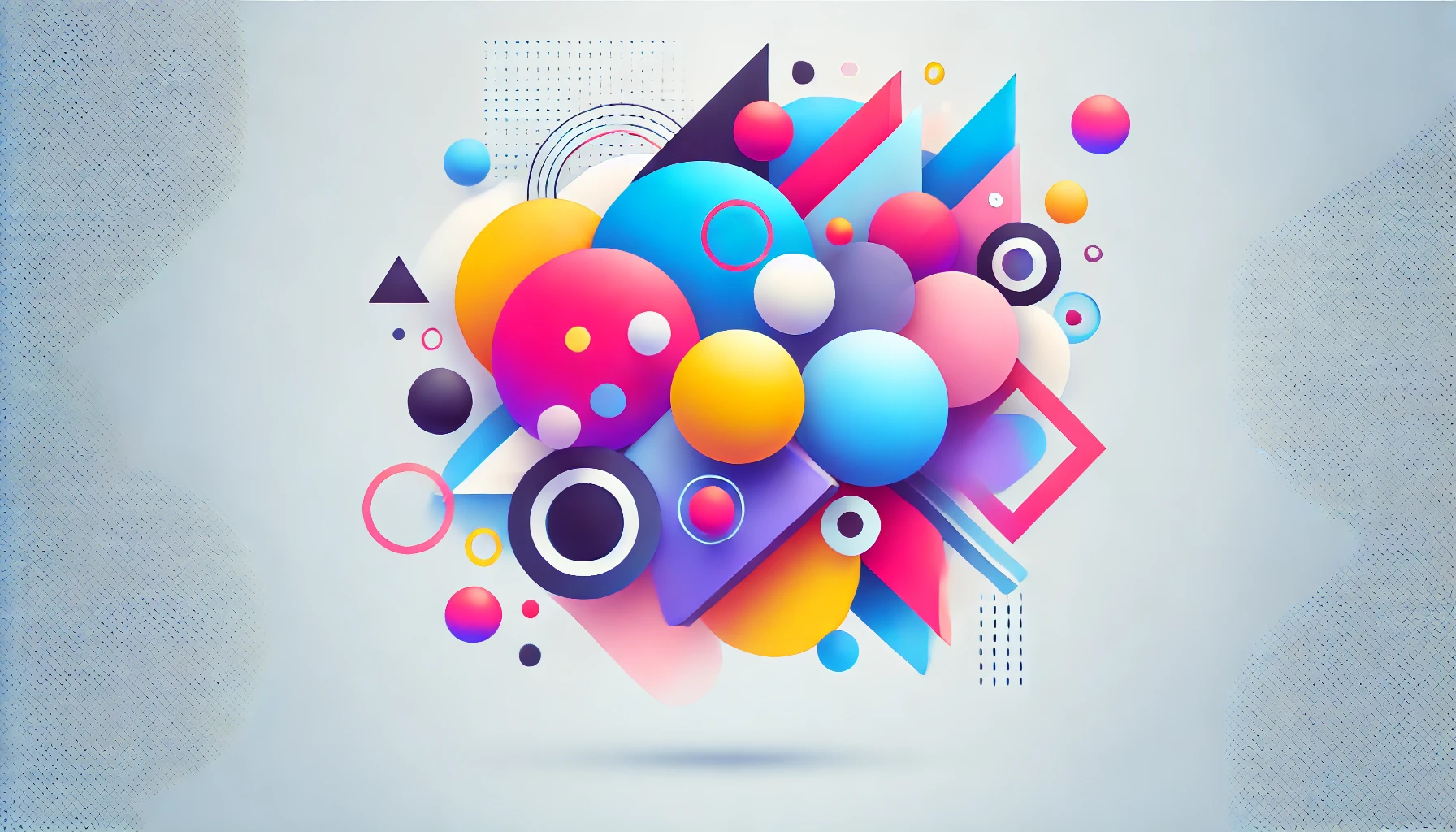
Safely Retrieve Properties Using Supplier and Optional in Java
The supplier allows you to pass something but not retrieve it right away. One use case is retrieving a property an object that could throw a null pointer exception. By not retrieving right away, but instead using a supplier, the supplier can be later executed inside a try/catch block and handle any exceptions thrown.