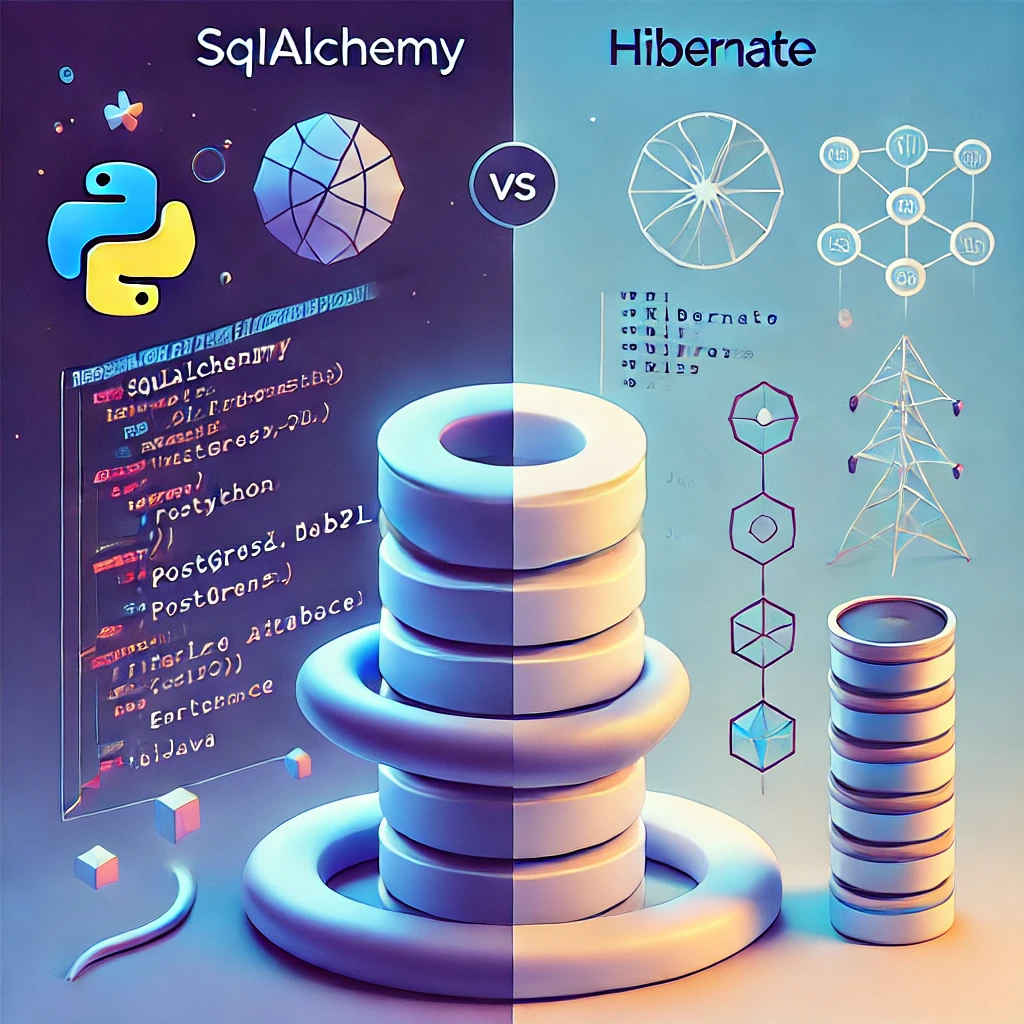
SQLAlchemy vs Hibernate: A Deep Dive Into Python and Java ORMs
Introduction
When developing applications that interact with databases, Object-Relational Mappers (ORMs) help bridge the gap between relational databases and object-oriented programming. Two of the most popular ORMs are SQLAlchemy (for Python) and Hibernate (for Java). While both serve the same purpose, they have different approaches, strengths, and best use cases.