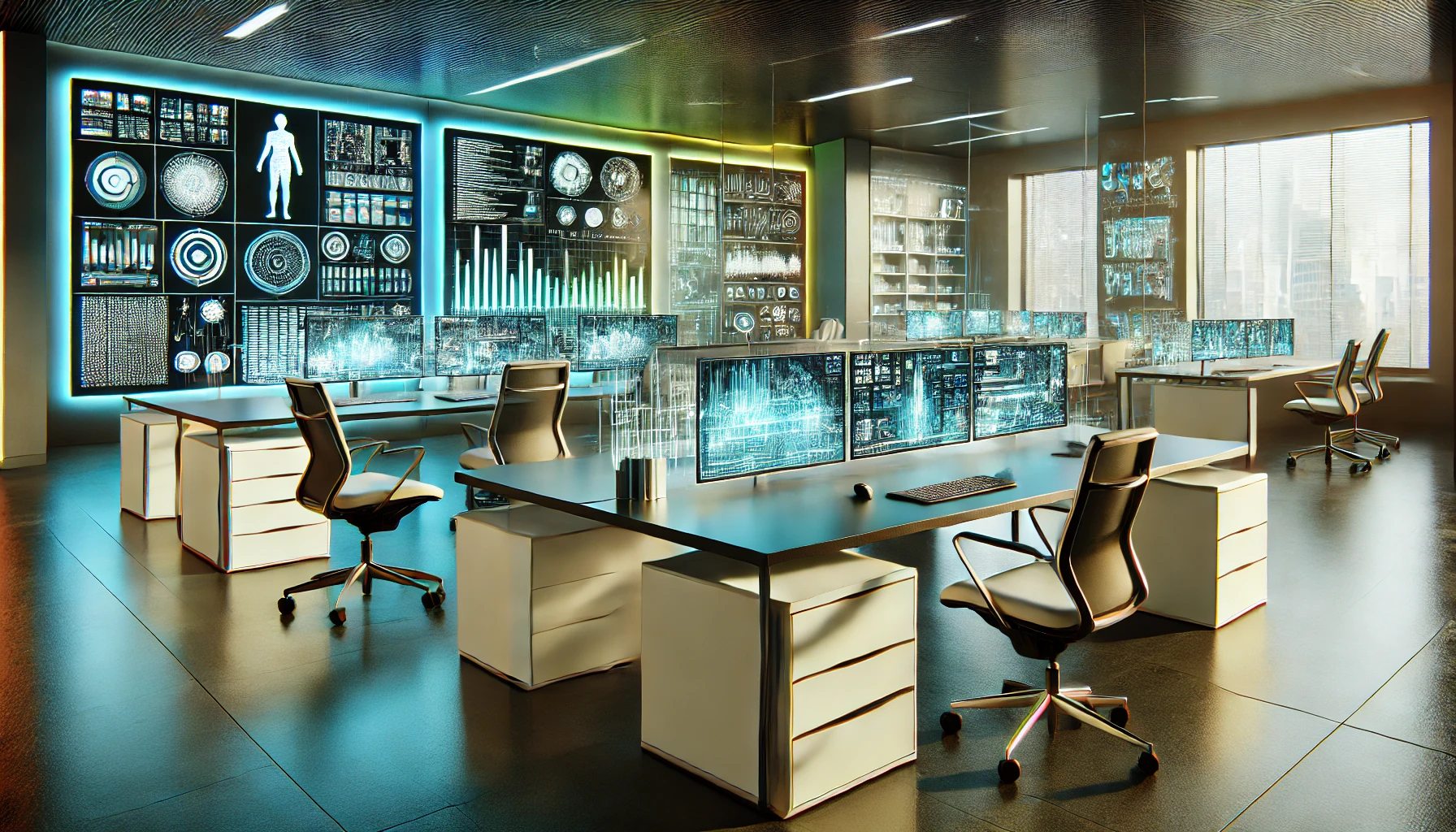
Function Composition in Java
An incredible tool in Java is the ability to create functions and compose them.
One common use case is to extract a variable from an object and then transform it.
In the object oriented paradigm, you would have a method that extracts and another that transforms. Then intermediate variables would be used to convert from the initial object to the final transformed result.
With function composition this is greatly simplified. The separate steps can be defined as functions and then composed together to create the chain of events that need to occur. Then, the final composed function can be used instead of manually calling each method. See the examples below.
Without function composition
private void withoutFunctionsWay(SomeObject initialObject) {
String strToTransform = extract(myObject);
String result = transform(strToTransform);
}
private String extract(SomeObject someObject) {
return someObject.getLevel1().getLevel2().getLevel3().getComplicatedLevel4().getVariable();
}
private String transform(String string) {
// Some sort of transformation
return string.toUpperCase();
}
With function composition
private void withFunctions(SomeObject initialObject) {
// Much cleaner, and this function can be pass around
String result = extractAndTransform.apply(initialObject);
}
Function<SomeObject, String> extract = someObject -> someObject.getLevel1().getLevel2().getLevel3().getComplicatedLevel4().getVariable();
Function<String, String> transform = str -> str.toUppercase();
Function<SomeObject, String> extractAndTransform = extract.andThen(transform);