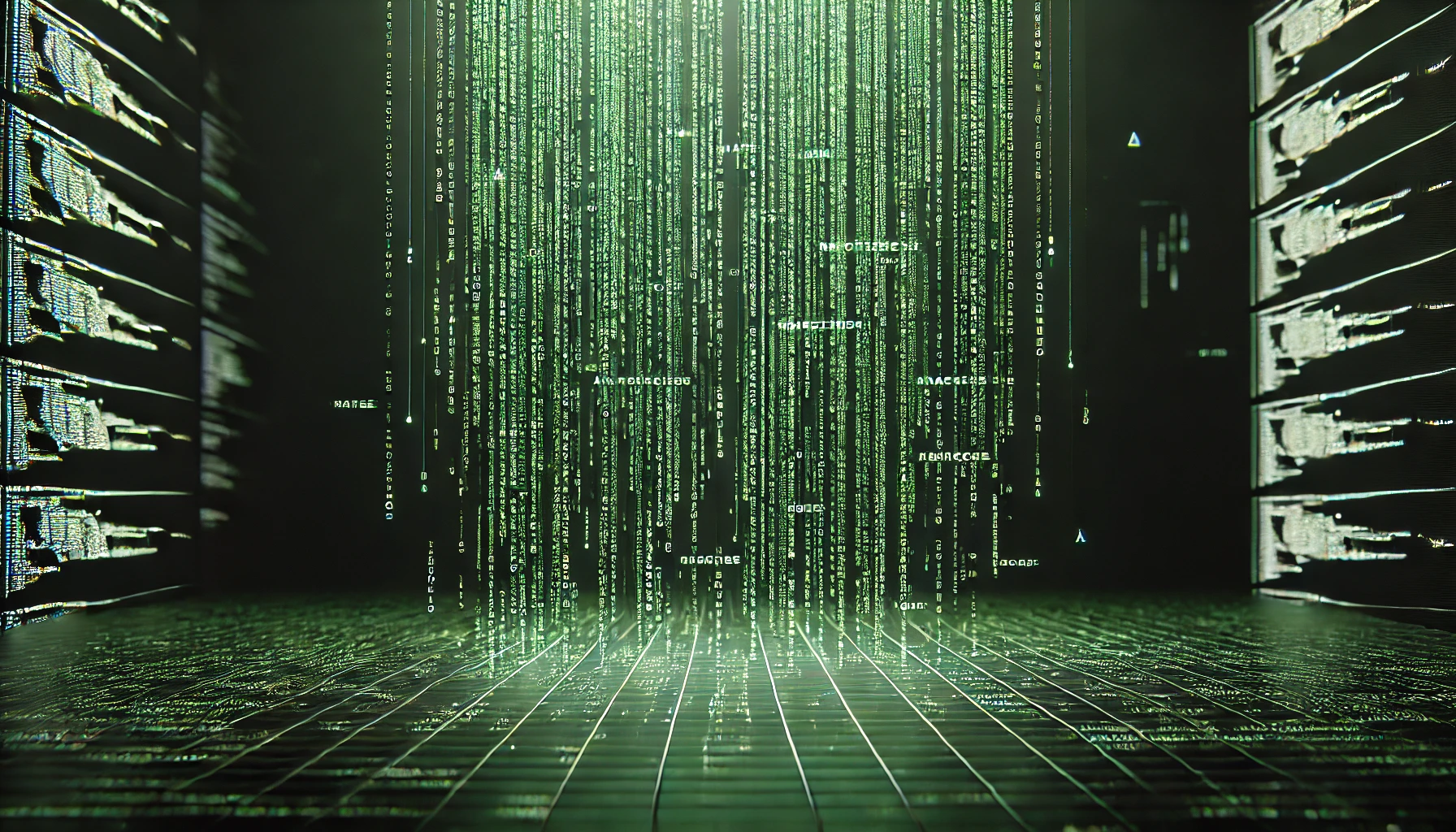
Recursion
Definition of Recursion:
Recursion is a programming technique where a function calls itself in order to solve smaller instances of the same problem until it reaches a base case that does not require further recursion.
Example in Python:
def factorial(n):
"""Calculate the factorial of a number using recursion."""
if n == 0:
return 1
else:
return n * factorial(n - 1)
# Example usage:
result = factorial(5)
print(f"The factorial of 5 is: {result}")
In this example, the factorial
function calculates the factorial of a number n
recursively. It calls itself with n - 1
until n
reaches 0 (base case).