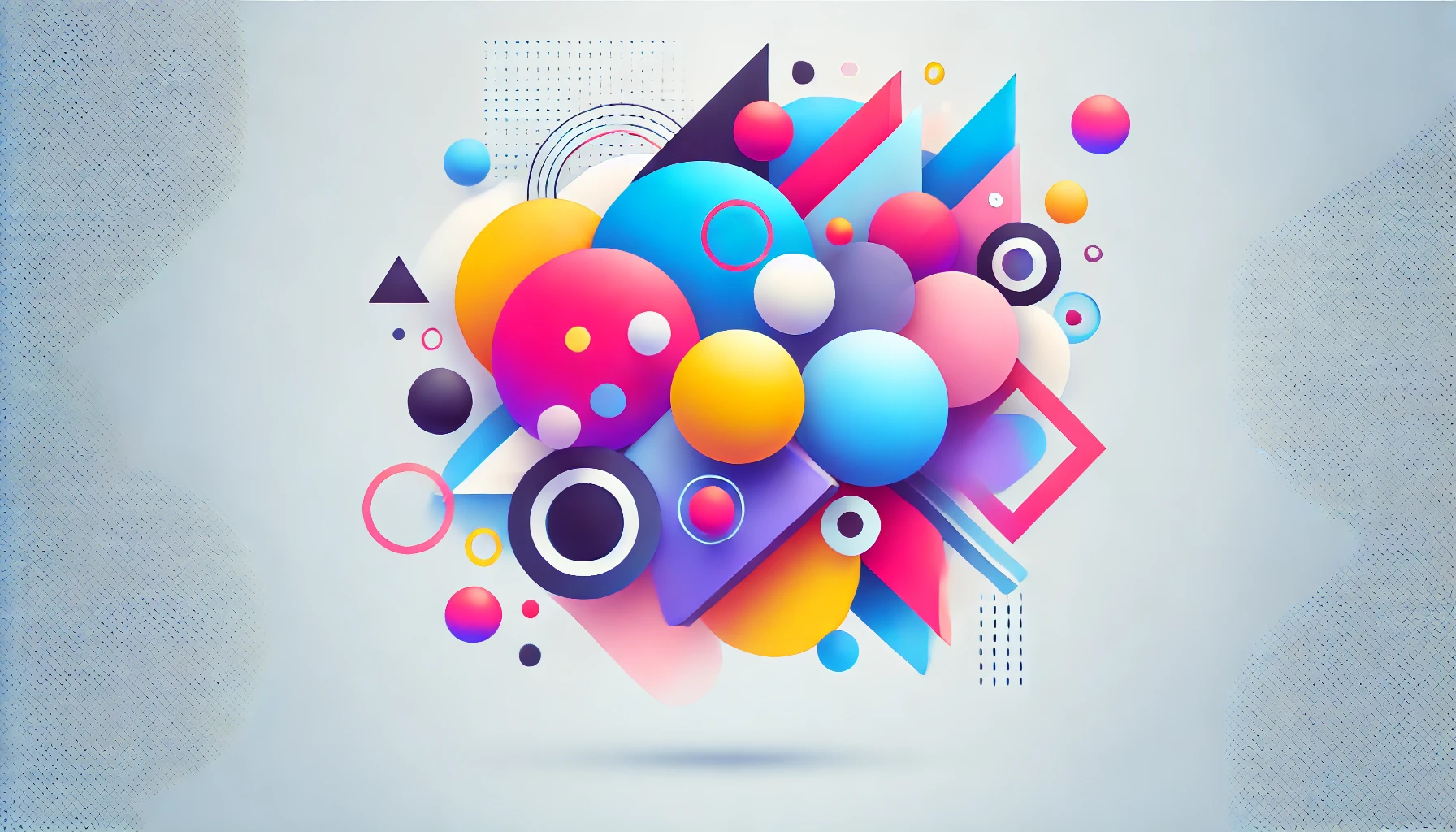
Safely retrieve properties using Supplier and Optional in Java
The supplier allows you to pass something but not retrieve it right away. One use case is retrieving a property an object that could throw a null pointer exception. By not retrieving right away, but instead using a supplier, the supplier can be later executed inside a try/catch block and handle any exceptions thrown.
public void example() {
// Without supplier - could throw a nullpointer exception on any getter
try {
String str = someObject.getLevel1().getLevel2().getLevel3().getString();
someOtherObject.appendValue(str);
catch (Exception e) {
// Exception throw - do nothing
// This code is ugly!!!
}
// With supplier
Optional<String> result = resolve() => someObject.getLevel1().getLevel2().getLevel3().getString());
// Now we can do something if the property is present or skip if it doesn't exist
// Much cleaner code
result.ifPresent(someOtherObject::appendValue);
}
// This resolve method can be reused
protected <T> Optional<T> resolve(Supplier<T> supplier) {
try {
T result = resolver.get();
return Optional.ofNullable(result);
} catch (NullPointerException) {
return Optional.empty();
}
}