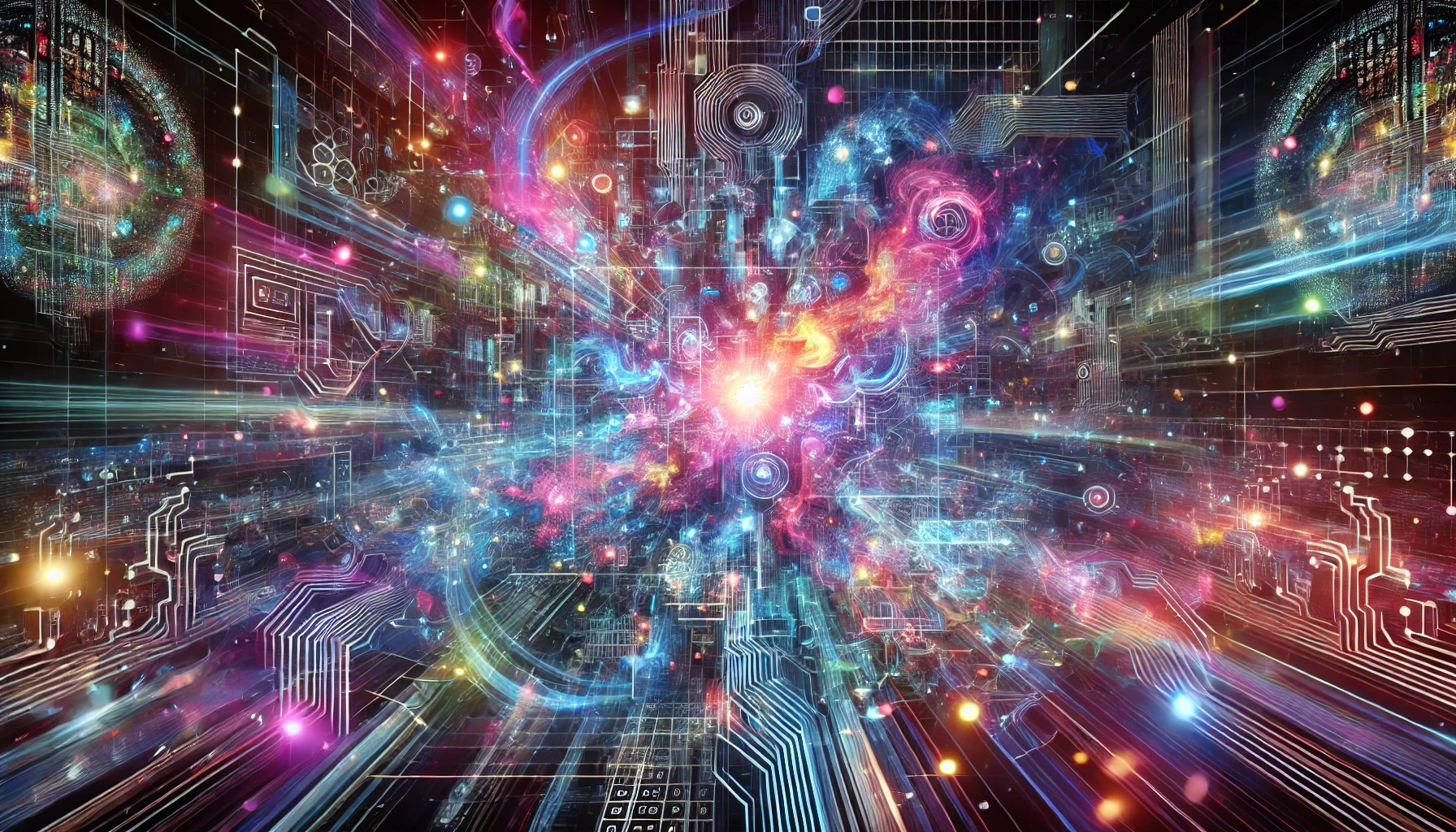
Set Interval Timer
Be careful when using setInterval as a timer.
let timeInSeconds = 0;
let interval = setInterval(() => {
someFunction();
timeInSeconds++;
}, 1000);
While it appears your time increments every second, it will actually increment one second (1000 milliseconds) plus the time the someFuntion() takes to run. This timer will eventually be out of sync.
A better approach is to create a point in time when the timer starts and then subtract the difference anytime the timeInSeconds is updated. In this scenerio, you can set the interval to any milliseconds amount and get an accurate time everytime the timeInSeconds variable is updated.
startTimer() {
this.startTime = Date.now()/1000;
let timeInSeconds = 0;
let interval = setInterval(() => {
someFunction();
timeInSeconds = Math.round((Date.now()/1000) - this.startTime); // Now displays actual seconds since started....
}, 1000);