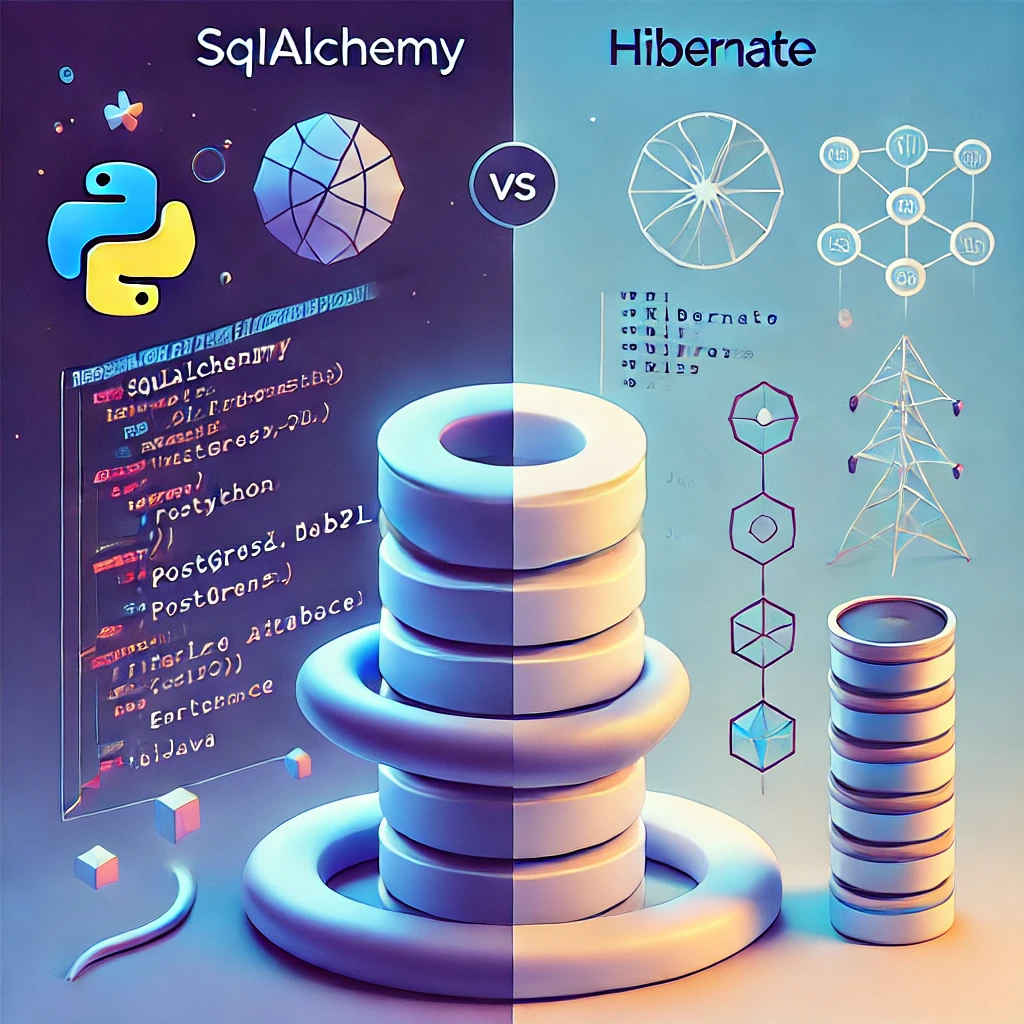
SQLAlchemy vs Hibernate: A Deep Dive into Python and Java ORMs
Introduction
When developing applications that interact with databases, Object-Relational Mappers (ORMs) help bridge the gap between relational databases and object-oriented programming. Two of the most popular ORMs are SQLAlchemy (for Python) and Hibernate (for Java). While both serve the same purpose, they have different approaches, strengths, and best use cases.
In this post, we’ll compare SQLAlchemy and Hibernate, provide code examples, and show how to generate SQLAlchemy classes from an existing PostgreSQL database using sqlacodegen
.
SQLAlchemy vs Hibernate: A Feature Comparison
Feature | SQLAlchemy (Python) | Hibernate (Java) |
---|---|---|
Language | Python | Java (also supports Kotlin, Scala, etc.) |
Querying Style | SQL Expression Language, ORM Query API | HQL (Hibernate Query Language), Criteria API |
Schema Generation | Manual or via sqlacodegen |
Auto-generated via annotations |
Lazy Loading | Yes | Yes (default) |
Caching | External libraries required | Built-in first-level and second-level caching |
Migrations | Alembic | Liquibase or Flyway |
Best Use Case | Python apps, data analytics, web apps | Enterprise Java applications, large-scale systems |
SQLAlchemy Example
SQLAlchemy provides both a low-level SQL Expression API and an ORM API. Here’s how you define models, insert, and query data using SQLAlchemy in Python.
1. Define Models
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker, relationship
# Database Connection
engine = create_engine('sqlite:///example.db', echo=True)
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String, unique=True)
posts = relationship("Post", back_populates="author")
class Post(Base):
__tablename__ = 'posts'
id = Column(Integer, primary_key=True)
title = Column(String)
content = Column(String)
user_id = Column(Integer, ForeignKey('users.id'))
author = relationship("User", back_populates="posts")
Base.metadata.create_all(engine)
2. Insert and Query Data
Session = sessionmaker(bind=engine)
session = Session()
new_user = User(name="Alice", email="alice@example.com")
session.add(new_user)
session.commit()
new_post = Post(title="My First Post", content="Hello, world!", user_id=new_user.id)
session.add(new_post)
session.commit()
user = session.query(User).filter_by(name="Alice").first()
print(f"User: {user.name}, Email: {user.email}")
Hibernate Example
Hibernate follows a Java-based ORM approach where entity classes are mapped using annotations.
1. Define Models in Java
import jakarta.persistence.*;
@Entity
@Table(name = "users")
class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String name;
private String email;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL)
private List<Post> posts;
}
@Entity
@Table(name = "posts")
class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String title;
private String content;
@ManyToOne
@JoinColumn(name = "user_id")
private User author;
}
2. Insert and Query Data in Hibernate
SessionFactory factory = new Configuration().configure("hibernate.cfg.xml").buildSessionFactory();
Session session = factory.openSession();
Transaction transaction = session.beginTransaction();
User user = new User("Alice", "alice@example.com");
session.persist(user);
transaction.commit();
Auto-generating SQLAlchemy Models from PostgreSQL using sqlacodegen
Instead of manually defining models, you can autogenerate them from an existing PostgreSQL database using sqlacodegen
.
1. Install Dependencies
pip install sqlalchemy psycopg2-binary sqlacodegen
2. Generate ORM Classes
sqlacodegen postgresql://username:password@localhost:5432/database_name --outfile models.py
3. Example Generated ORM Model
from sqlalchemy import Column, Integer, String, ForeignKey
from sqlalchemy.orm import relationship, declarative_base
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String, unique=True)
posts = relationship('Post', back_populates='author')
class Post(Base):
__tablename__ = 'posts'
id = Column(Integer, primary_key=True)
title = Column(String)
content = Column(String)
user_id = Column(Integer, ForeignKey('users.id'))
author = relationship('User', back_populates='posts')
Conclusion
Both SQLAlchemy and Hibernate are powerful ORMs, but their best use cases differ:
- Use SQLAlchemy for Python applications, especially in web development and data-driven projects.
- Use Hibernate for enterprise Java applications where scalability and caching are critical.
If working with an existing PostgreSQL database in SQLAlchemy, using sqlacodegen
can save time and effort when defining models.